The code you will find next is an adaptation of Chris Dahms original License Plate Recognition. A video of his work can be found here as longs as his original source code. I made this work (pretty much some refactoring) mainly because I was in need of a simple system to detect the license plate area in images. Code is in Python 3.6. Feb 18, 2015 In this tutorial I show how to use the Tesseract - Optical Character Recognition (OCR) in conjunction with the OpenCV library to detect text on a license plate recognition application. Automatic License Plate Recognition using Python and OpenCV K.M. Sajjad Department of Computer Science and Engineering M.E.S. College of Engineering, Kuttippuram, Kerala me@sajjad.in Abstract—Automatic License Plate Recognition system is a real time embedded system which automatically recognizes the license plate of vehicles.
OpenALPR is an open source Automatic License Plate Recognition library written in C++ with bindings in C#, Java, Node.js, Go, and Python. The library analyzes images and video streams to identify license plates. The output is the text representation of any license plate characters.
Check out a live online demo here: http://www.openalpr.com/demo-image.html
User Guide
OpenALPR includes a command line utility. Simply typing 'alpr [image file path]' is enough to get started recognizing license plate images.
For example, the following output is created by analyzing this image:
Detailed command line usage:
Binaries
Pre-compiled Windows binaries can be downloaded on the releases page
Install OpenALPR on Ubuntu 16.04 with the following commands:
Documentation
Detailed documentation is available at doc.openalpr.com
Integrating the Library
OpenALPR is written in C++ and has bindings in C#, Python, Node.js, Go, and Java. Please see this guide for examples showing how to run OpenALPR in your application: http://doc.openalpr.com/bindings.html
Compiling
OpenALPR compiles and runs on Linux, Mac OSX and Windows.
OpenALPR requires the following additional libraries:
After cloning this GitHub repository, you should download and extract Tesseract and OpenCV source code into their own directories. Compile both libraries.
Please follow these detailed compilation guides for your respective operating system:
If all went well, there should be an executable named alpr along with libopenalpr-static.a and libopenalpr.so that can be linked into your project.
Docker
Questions
Please post questions or comments to the Google group list: https://groups.google.com/forum/#!forum/openalpr
Contributions
Improvements to the OpenALPR library are always welcome. Please review the OpenALPR design description and get started.
Code contributions are not the only way to help out. Do you have a large library of license plate images? If so, please upload your data to the anonymous FTP located at upload.openalpr.com. Do you have time to 'tag' plate images in an input image or help in other ways? Please let everyone know by posting a note in the forum.
License
Affero GPLv3http://www.gnu.org/licenses/agpl-3.0.html
Commercial-friendly licensing available. Contact: info@openalpr.com
I am facing a problem in segmenting characters from a license plate image.I have applied following method to extract license plate characters'
- Adaptive threshold the license plate image.
- Select contours which having particular aspect ratio.
If there is any shade in the license plate image as in attached file, I am not able to properly segment the characters due to improper binarization. The shade in the image merges adjacent characters in the image.
I have thresholded the images with different window sizes. The results are attached. How can I segment characters from image if there is shade in the image? I am using OpenCV.
I have used following function in OpenCV to threshold my license plate image:
I have tried with different window sizes (wind
) and different adaptiveMethod
(ADAPTIVE_THRESH_MEAN_C and ADAPTIVE_THRESH_GAUSSIAN_C
)to get the thresholded images.
2 Answers
Before I start, I know you are seeking an implementation of this algorithm in OpenCV C++, but my algorithm requires the FFT and the numpy / scipy
packages are awesome for that. As such, I will give you an implementation of the algorithm in OpenCV using Python instead. The code is actually quite similar to the C++ API that you can easily transcribe that over instead. That way, it minimizes the amount of time it will take for me to learn (or rather relearn...) the API and I would rather give you the algorithm and the steps I did to perform this task to not waste any time at all.
Opencv Python License Plate Search
As such, I will give you a general overview of what I would do. I will then show you Python code that uses numpy, scipy
and the OpenCV packages. As a bonus for those who use MATLAB, I will show you the MATLAB equivalent, with MATLAB code to boot!
What you can do is try to use homomorphic filtering. In basic terms, we can represent an image in terms of a product of illumination and reflectance. Illumination is assumed to be slowly varying and the main contributor of dynamic range. This is essentially low frequency content. Reflectance represents details of objects and assumed to vary rapidly. This is also the primary contributor to local contrast and is essentially high frequency content.
The image can be represented as a product of these two. Homomorphic filtering tries and splits up these components and we filter them individually. We then combine the results together when we are finished. As this is a multiplicative model, it's customary to use a log operation so that we can express the product as a sum of two terms. These two terms are filtered individually, scaled to emphasize or de-emphasize their contributions to the image, summed, then the anti-log is taken.
The shading is due to the illumination, and so what we can do is decrease the contribution that this shading does over the image. We can also boost the reflectance so we can get some better edges as edges are associated with high frequency information.
We usually filter the illumination using a low-pass filter, while the reflectance with a high-pass filter. In this case, I'm going to choose a Gaussian kernel with a sigma of 10 as the low-pass filter. A high-pass filter can be obtained by taking 1
and subtracting with the low-pass filter. I transform the image into the log domain, then filter the image in the frequency domain using the low-pass and high-pass filters. I then scale the low pass and high pass results, add these components back, then take the anti-log. This image is now better suited to be thresholded as the image has low variation.
What I do as additional post-processing is that I threshold the image. The letters are darker than the overall background, so any pixels that are lower than a certain threshold would be classified as text. I chose the threshold to be intensity 65. After this, I also clear off any pixels that are touching the border, then remove any areas of the image that have less than 160 (MATLAB) or 120 (Python) pixels of total area. I also crop out some of the columns of the image as they are not needed for our analysis.
Here are a couple of caveats for you:
Caveat #1 - Removing borders
Removing any pixels that touch the border is not built into OpenCV. However, MATLAB has an equivalent called imclearborder
. I'll use this in my MATLAB code, but for OpenCV, this was the following algorithm:
- Find all of the contours in the image
- For each contour that is in the image, check to see if any of the contour pixels are within the border of the image
- If any are, mark this contour for removal
- For each contour we want to remove, simply draw this whole contour in black
I created a method called imclearborder(imgBW, radius)
in my code, where radius
is how many pixels within the border you want to clear stuff up.
Caveat #2 - Removing pixel areas below a certain area
Removing any areas where they are less than a certain amount is also not implemented in OpenCV. In MATLAB, this is conveniently given using bwareaopen
. The basic algorithm for this is:
- Find all of the contours in the image
- Analyze how much each contour's area fills up if you were to fill in the interior
- Any areas that are less than a certain amount, clear this contour by filling the interior with black
I created a method called bwareaopen(imgBW)
that does this for us.
Caveat #3 - Area parameter for removing pixel areas

For the Python code, I had to play around with this parameter and I settled for 120. 160 was used for MATLAB. For python, 120 got rid of some of the characters, which is not desired. I'm guessing my implementation of bwareaopen
in comparison to MATLAB's is different, which is probably why I'm getting different results.
Without further ado, here's the code. Take note that I did not use spatial filtering. You could use filter2D
in OpenCV and convolve this image with the Gaussian kernel, but I did not do that as Homomorphic Filtering when using low-pass and high-pass filters are traditionally done in the frequency domain. You could explore this using spatial filtering, but you would also have to know the size of your kernels before hand. With frequency domain filtering, you just need to know the standard deviation of the filter, and that's just one parameter in comparison to two.
Also, for the Python code, I downloaded your image on to my computer and ran the script. For MATLAB, you can directly reference the hyperlink to the image when reading it in with the Image Processing toolbox.
This is the result I get:
Take note that I re-arranged the windows so that they're aligned in a single column.
rayryeng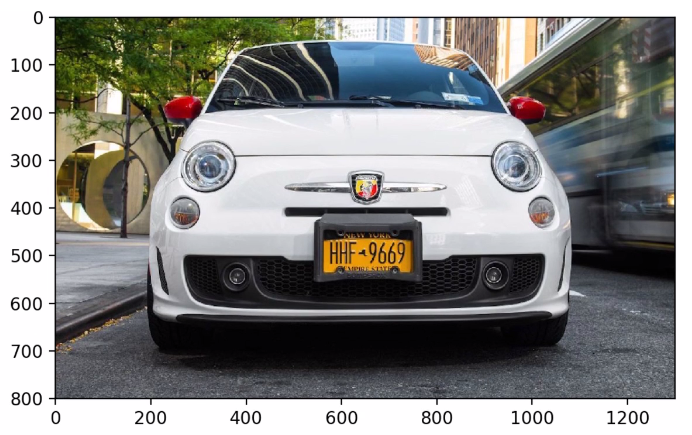
I think you will get a good image if you apply a morphological opening operation to the second binarized image that you have provided.